When you first step into the world of Arduino, the blinking LED project is the quintessential starting point. It’s simple, fun, and incredibly rewarding. But why is it called the “Hello World” of Arduino? Because just like writing “Hello World” in a new programming language, making an LED blink is the first tangible proof that you’ve successfully programmed your microcontroller. Let’s dive into the blinking LED project, explore its significance, and learn how to build it step by step.
Why Start with a Blinking LED?
Starting simple is key when learning any new skill, and Arduino is no exception. The blinking LED project allows beginners to familiarize themselves with basic coding, circuit building, and how the Arduino ecosystem works.
Think of it like learning to ride a bike with training wheels. You won’t win any races, but you’ll understand the fundamentals and build confidence.
What You’ll Need
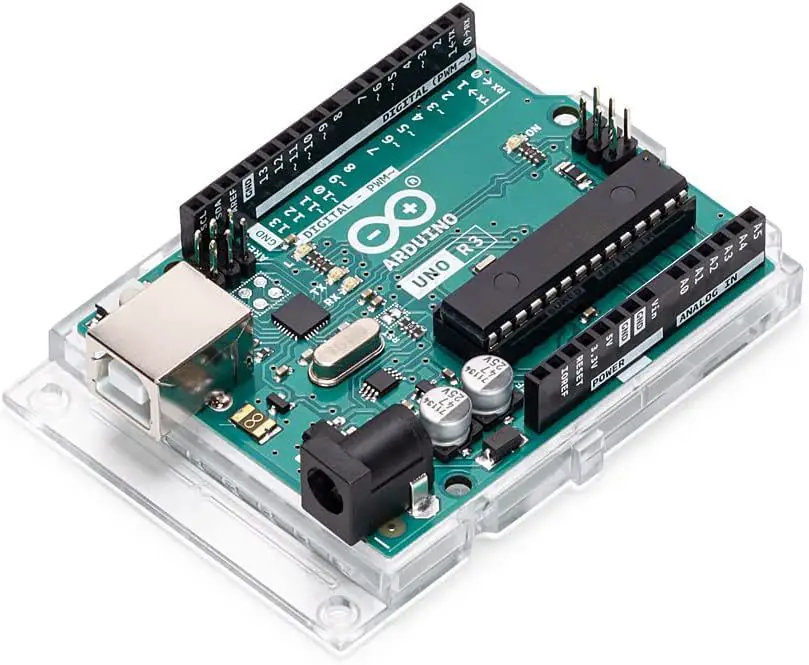
Before we begin, let’s gather the materials. Fortunately, the blinking LED project requires only a few components, most of which are included in any Arduino starter kit.
Read Also Automatic Plant Watering Project With Smart Phone Control
Components List:
- Arduino Board: Uno, Nano, or Mega will work perfectly.
- LED: A basic 5mm LED.
- Resistor: A 220-ohm or 1kilo-ohms resistor to limit the current.
- Breadboard: For easy circuit connections.
- Jumper Wires: To connect components. You can use a male-male jumper wire type or preformed jumper wires.
- USB Cable: To upload the code and power your Arduino.
Setting Up Your Workspace
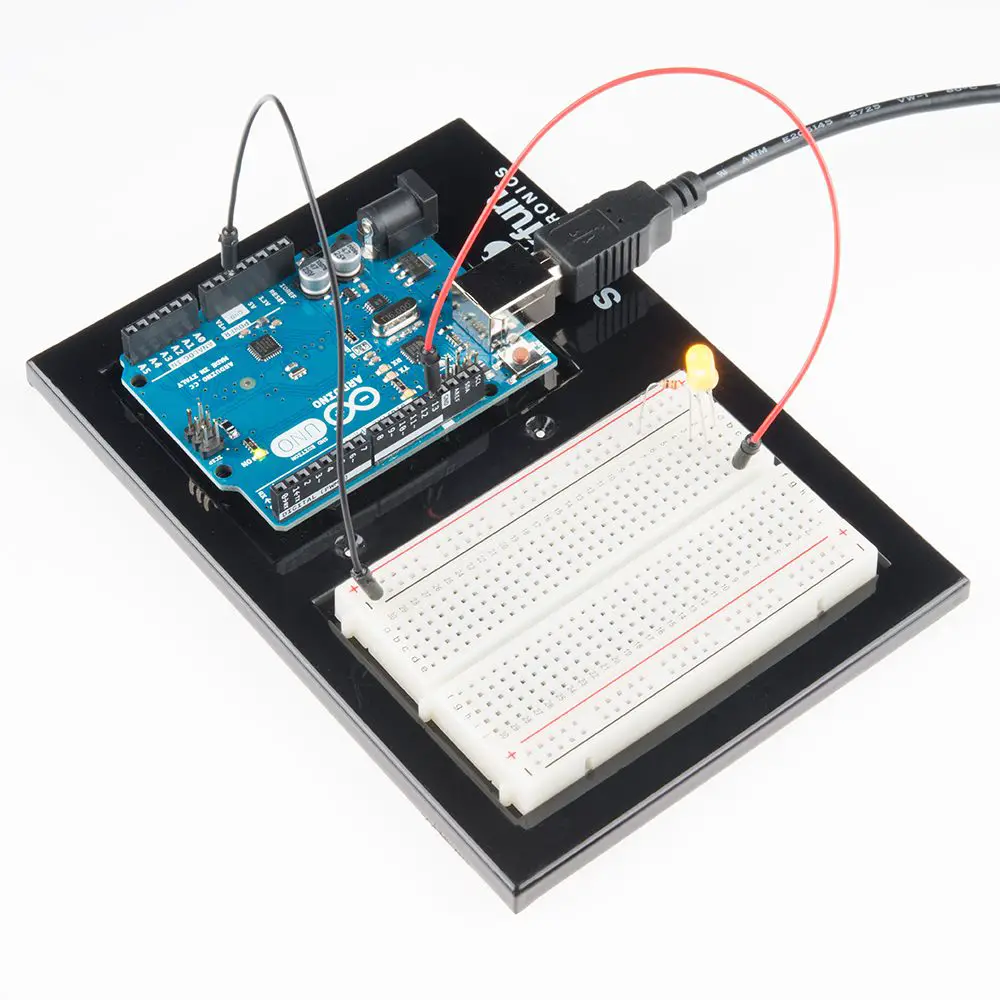
To build this project, you’ll need a tidy and safe workspace. Avoid clutter and ensure you have adequate lighting. Keep your laptop and Arduino IDE (Integrated Development Environment) ready.
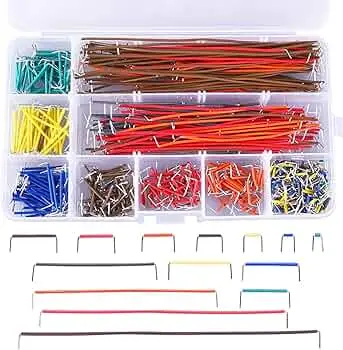
Pro tip: If you’re new to using breadboards, take a moment to understand how the rows and columns are connected internally. It’ll save you troubleshooting time later.
Circuit Diagram
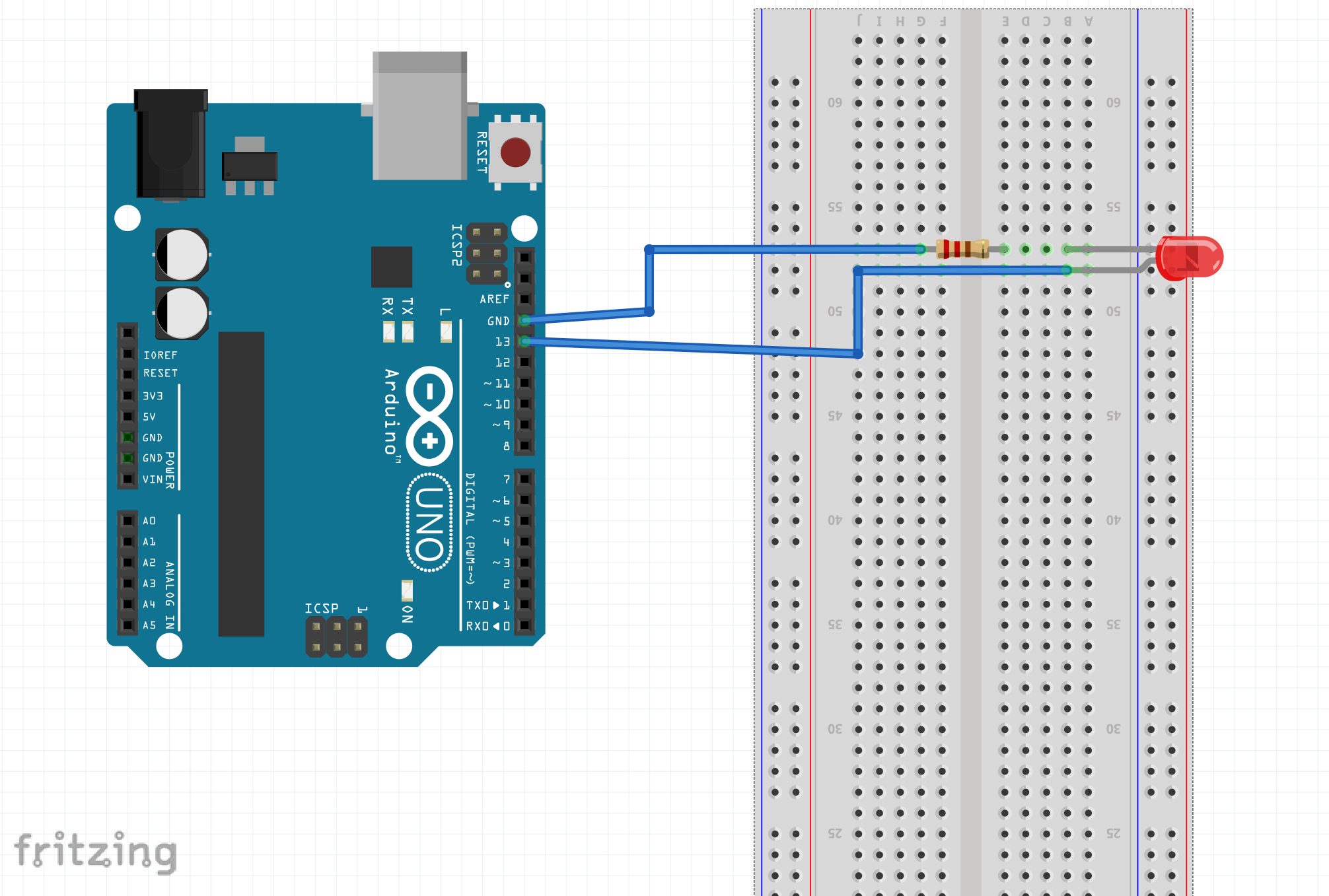
Explanation of Circuit Diagram
The blinking LED circuit is as simple as it gets. Here’s how to connect the components:
- Connect the LED: Insert the LED into the breadboard. The longer leg (anode) is the positive terminal, while the shorter leg (cathode) is the negative terminal.
- Add the Resistor: Connect one end of the resistor to the anode of the LED and the other end to a jumper wire.
- Link to Arduino: Attach the resistor’s free end to digital pin 13 on the Arduino board. Connect the cathode of the LED to the Arduino’s GND (ground) pin.
- Power Up: Use the USB cable to connect your Arduino to your computer.
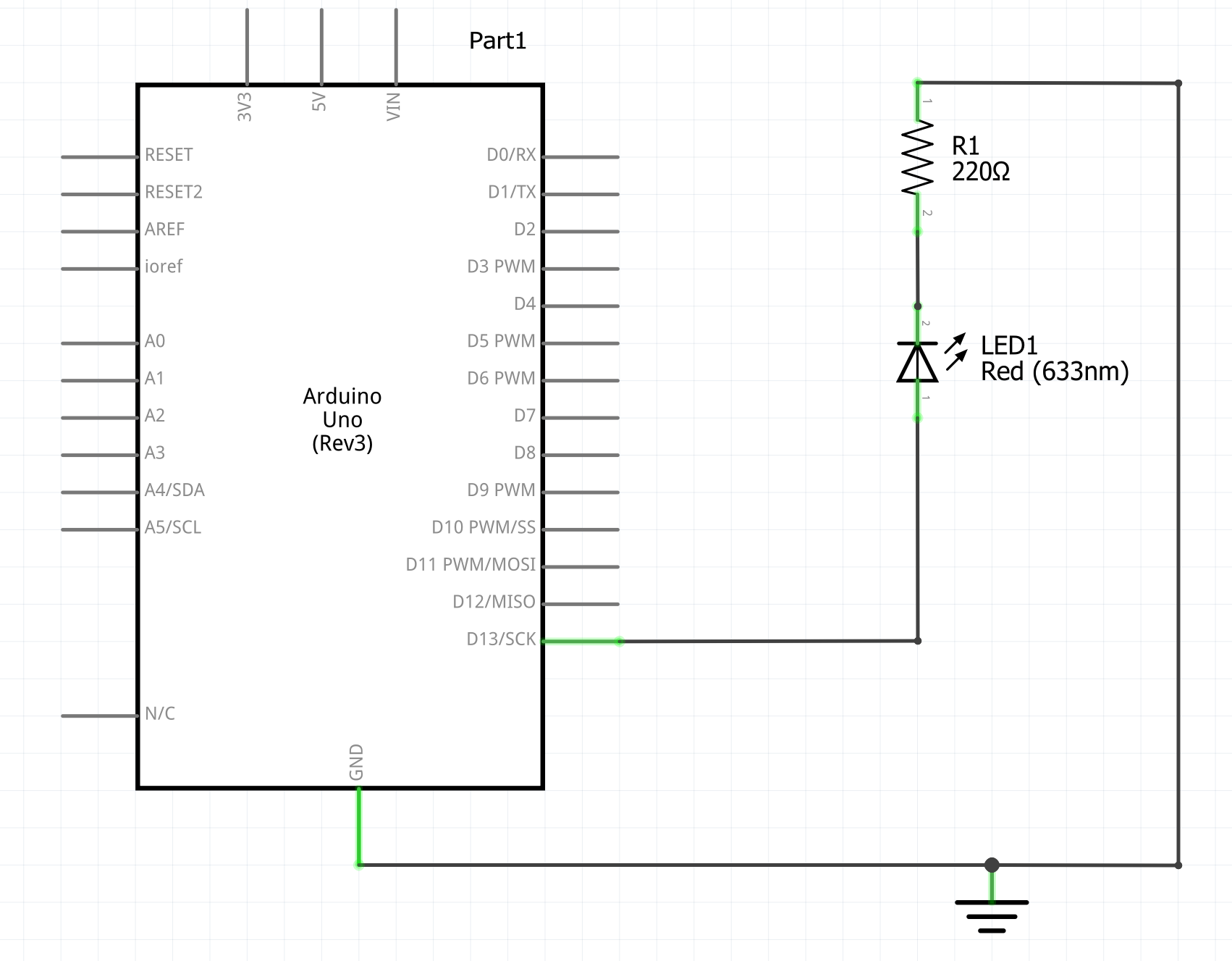
Writing The Arduino Code (Sketch)
With the hardware ready, let’s move on to coding. Open the Arduino IDE and create a new sketch. The blinking LED code is surprisingly straightforward.
void setup() {
pinMode(13, OUTPUT); // Set pin 13 as an output
}
void loop() {
digitalWrite(13, HIGH); // Turn the LED on
delay(1000); // Wait for 1 second
digitalWrite(13, LOW); // Turn the LED off
delay(1000); // Wait for 1 second
}
Understanding the Code
pinMode(13, OUTPUT)
: Configures pin 13 to send an electrical signal.digitalWrite
: Controls the state of the pin—HIGH
turns the LED on, andLOW
turns it off.delay(1000)
: Pauses the program for 1 second (1000 milliseconds).
Uploading the Code
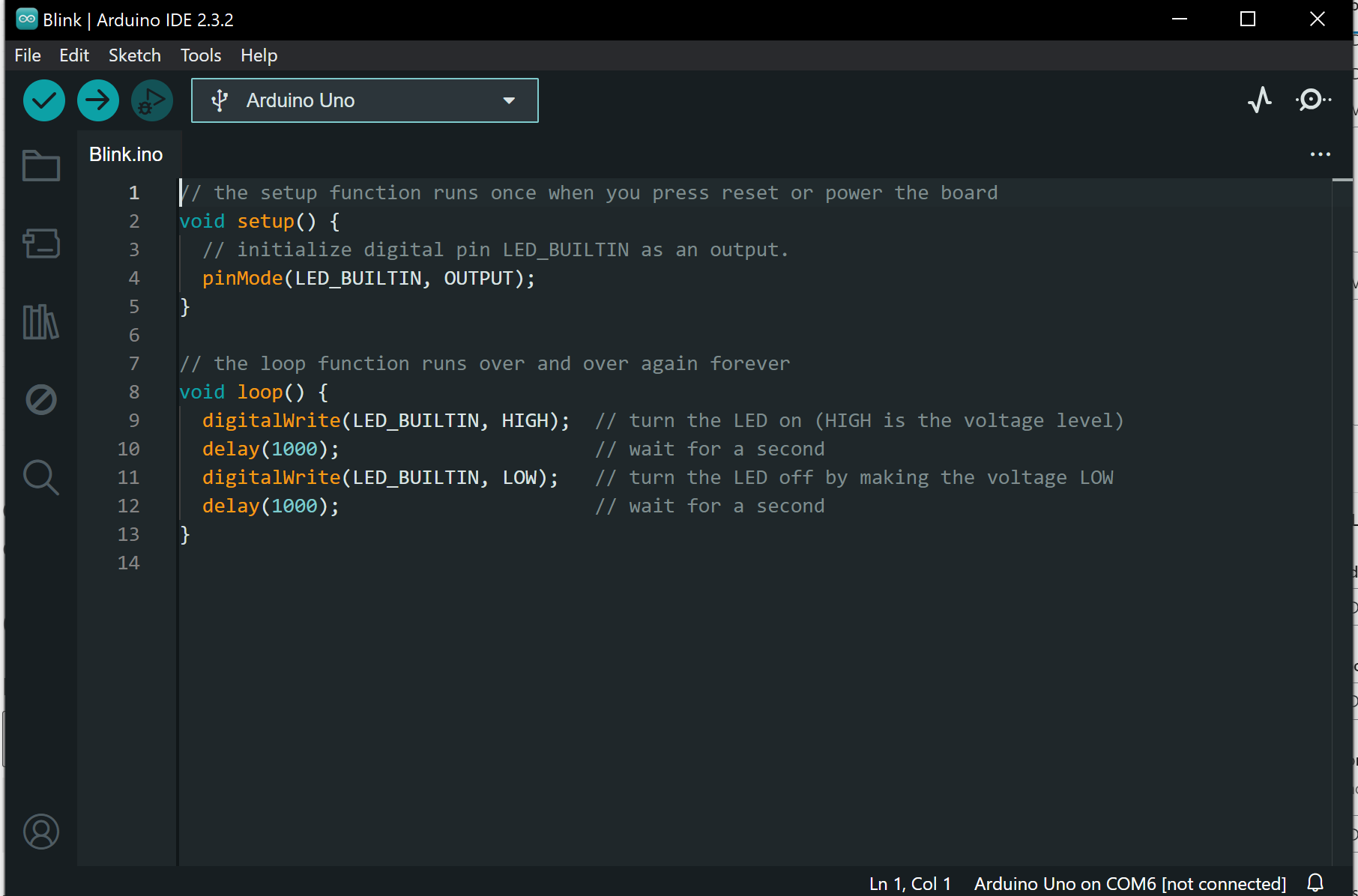
- Connect your Arduino to your computer via USB.
- Select the correct board and port in the Arduino IDE under Tools.
- Click the Upload button.
If everything is set up correctly, you’ll see the LED blink on and off at one-second intervals. Congratulations, you’ve just built your first Arduino project!
Experimenting with Blinking Patterns
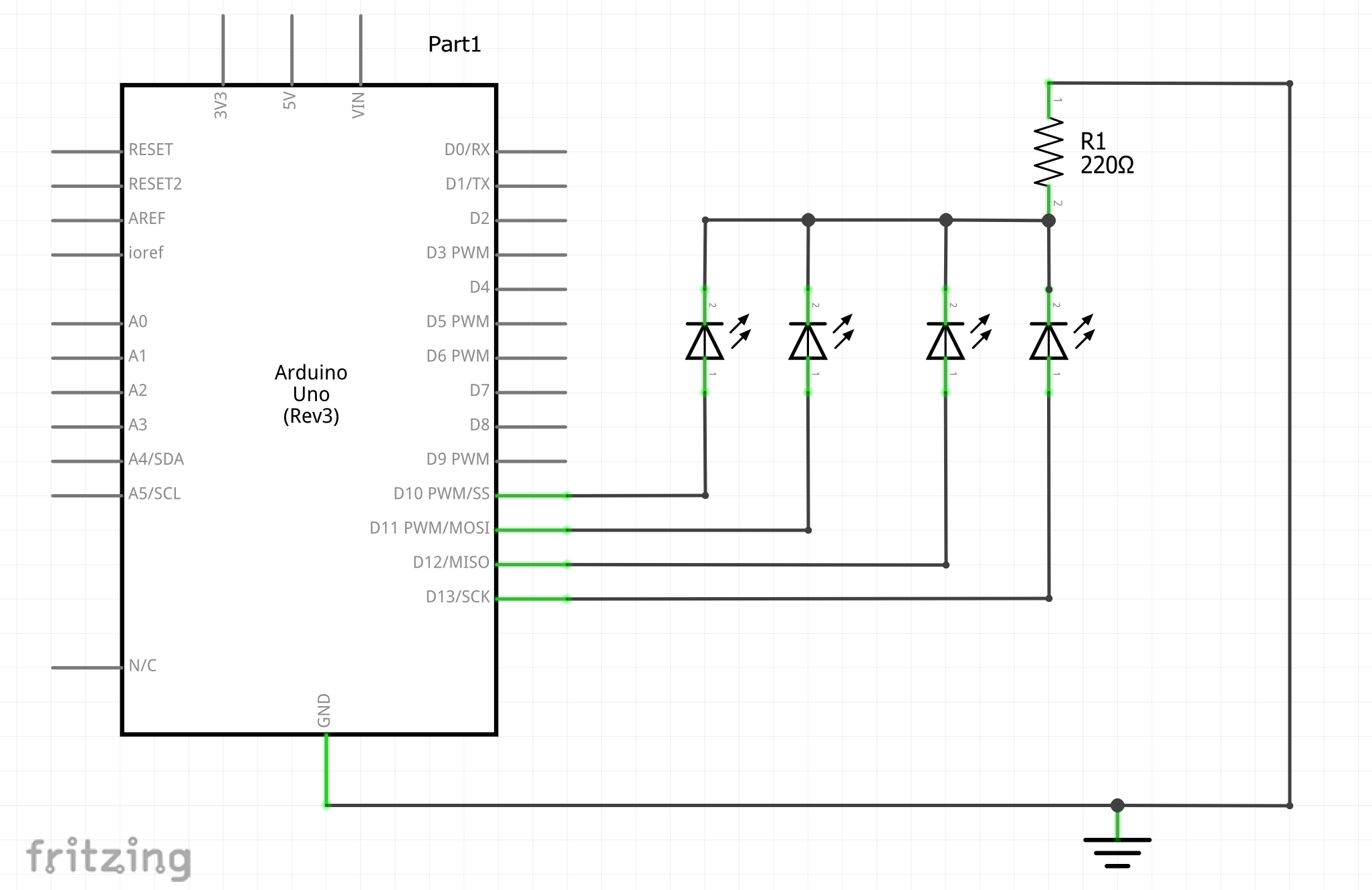
Why stop at a single blink? Here are some ways to experiment with your code. But first connect more LEDs as shown in the circuit diagram above.
Speed It Up
Change the delay value to 500 milliseconds for a faster blink rate.
delay(500);
Add More LEDs
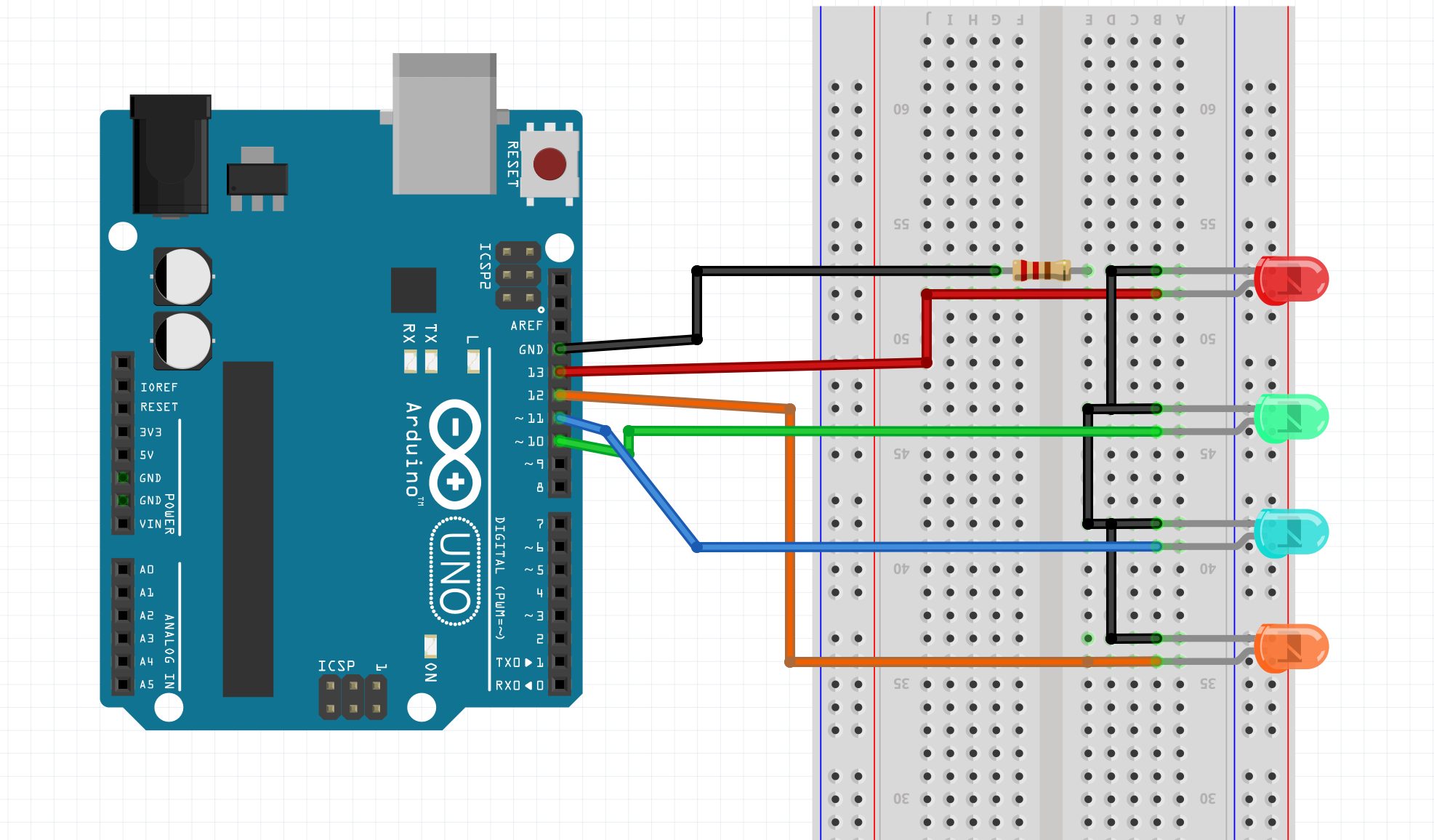
Connect additional LEDs to other pins (e.g., pin 12 or 11) and control them with separate digitalWrite
commands.
Create a SOS Signal
Mimic the Morse code for SOS (short-short-short, long-long-long, short-short-short) by adjusting the delay
values.
#define t 30
#define t1 20
#define t2 100
#define t3 50
#define max_led 5
int ledpins[5] = {27, 16, 2, 16, 26};
void setup() {
// set up pins 2 to 13 as outputs
for (int i = 0; i <= max_led; i++) {
pinMode(ledpins[i], OUTPUT);
}
}
// function to switch all LEDs off
void clear(void)
{
for (int i = 0; i <= max_led; i++) {
digitalWrite(i, LOW);
}
}
/////////////////////////////////////////////////////////////////////////////////Effect 1
//left to right and right to left
void effect_1()
{
for(int i=0; i<max_led; i++){
digitalWrite(ledpins[i], HIGH);
delay(t1);
digitalWrite(ledpins[i+1], HIGH);
delay(t1);
digitalWrite(ledpins[i+2], HIGH);
delay(t1);
digitalWrite(ledpins[i], LOW);
delay(t1);
digitalWrite(ledpins[i+1], LOW);
delay(t1);
}
for(int i=max_led-1; i>0; i--){
digitalWrite(ledpins[i], HIGH);
delay(t1);
digitalWrite(ledpins[i-1], HIGH);
delay(t1);
digitalWrite(ledpins[i-2], HIGH);
delay(t1);
digitalWrite(ledpins[i], LOW);
delay(t1);
digitalWrite(ledpins[i-1], LOW);
delay(t1);
}
}
/////////////////////////////////////////////////////////////////////////////////Effect 2
void effect_2()
{
int count = max_led-1; // keeps track of second LED movement
// move first LED from left to right and second from right to left
for (int i = 0; i < max_led; i++) {
clear();
digitalWrite(ledpins[i], HIGH); // chaser 1
digitalWrite(ledpins[count], HIGH); // chaser 2
count--;
// stop LEDs from appearing to stand still in the middle
if (count != 2) {
delay(t2);
}
}
// move first LED from right to left and second LED from left to right
for (int i = max_led-1; i > 0; i--) {
clear();
digitalWrite(ledpins[i], HIGH); // chaser 1
digitalWrite(ledpins[count], HIGH); // chaser 2
count++;
// stop LEDs from appearing to stand still in the middle
if (count != 2) {
delay(t2);
}
}
}
////////////////////////////////////////////////////////////////////////////////Effect 3
void effect_3()
{
for(int i=0; i<max_led; i++){
digitalWrite(ledpins[i], HIGH);
delay(t3);
}
for(int i=0; i<max_led; i++){
digitalWrite(ledpins[i], LOW);
delay(t3);
}
for(int i = max_led-1; i>=0; i--){
digitalWrite(ledpins[i], HIGH);
delay(t3);
}
for(int i = max_led-1; i>=0; i--){
digitalWrite(ledpins[i], LOW);
delay(t3);
}
}
///////////////////////////////////////////////////////////////////////////Effect 4
void effect_4()
{
for(int j = 0; j <= max_led; j++){
digitalWrite(ledpins[j], HIGH);
delay(t2);
j=j+1;
}
for(int j = 0; j <= max_led; j++){
digitalWrite(ledpins[j], LOW);
delay(t2);
}
for(int k = max_led; k > 0; k--){
digitalWrite(ledpins[k], HIGH);
delay(t2);
k=k-1;
}
for(int k = max_led; k > 0; k--){
digitalWrite(ledpins[k], LOW);
delay(t2);
}
}
//////////////////////////////////////////////////////////////////////////////Effect 5
void effect_5()
{
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(ledpins[pin], HIGH);
delay(t1);
digitalWrite(ledpins[pin+1], LOW);
delay(t1);
}
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(ledpins[pin+1], HIGH);
delay(t1);
digitalWrite(ledpins[pin+2], LOW);
delay(t1);
}
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(ledpins[pin+2], HIGH);
delay(t1);
digitalWrite(ledpins[pin+3], LOW);
delay(t1);
}
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(ledpins[pin+3], HIGH);
delay(t1);
digitalWrite(ledpins[pin+4], LOW);
delay(t1);
}
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(ledpins[pin+4], HIGH);
delay(t1);
digitalWrite(ledpins[pin+5], LOW);
delay(t1);
}
/*
for(int pin = max_led-1; pin >= 0; pin--)
{
digitalWrite(pin+5, HIGH);
delay(t1);
digitalWrite(pin+6, LOW);
delay(t1);
}
for(int pin = 13; pin >= 2; pin--)
{
digitalWrite(pin+6, HIGH);
delay(t1);
digitalWrite(pin+7, LOW);
delay(t1);
}
for(int pin = 13; pin >= 2; pin--)
{
digitalWrite(pin+7, HIGH);
delay(t1);
digitalWrite(pin+8, LOW);
delay(t1);
}
for(int pin = 9; pin >= 2; pin--)
{
digitalWrite(pin+8, HIGH);
delay(t1);
digitalWrite(pin+9, LOW);
delay(t1);
}
for(int pin = 9; pin >= 2; pin--)
{
digitalWrite(pin+9, HIGH);
delay(t1);
digitalWrite(pin+10, LOW);
delay(t1);
}
for(int pin = 9; pin >= 2; pin--)
{
digitalWrite(pin+10, HIGH);
delay(t1);
digitalWrite(pin+11, LOW);
delay(t1);
}
for(int pin = 14; pin >= 2; pin--)
{
digitalWrite(pin+11, HIGH);
delay(t1);
}
*/
for(int pin = 13; pin >= 2; pin--)
{
digitalWrite(pin, LOW);
delay(t1);
}
}
/////////////////////////////////////////////////////////////////////////////Effect 6
void effect_6()
{
for(int j=0; j<max_led; j++){
digitalWrite(ledpins[j], LOW);
delay(t);
digitalWrite(ledpins[j], HIGH);
delay(t);
digitalWrite(ledpins[j-2], LOW);
delay(t);
digitalWrite(ledpins[j], HIGH);
}
for(int k = max_led; k>0; k--){
digitalWrite(ledpins[k], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
delay(t);
digitalWrite(ledpins[k+2], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
}
for(int k = 0; k<max_led; k++){
digitalWrite(ledpins[k], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
delay(t);
digitalWrite(ledpins[k-2], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
}
for(int k = max_led+1; k>0; k--){
digitalWrite(ledpins[k], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
delay(t);
digitalWrite(ledpins[k+4], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
}
for(int k = 0; k<max_led+1; k++){
digitalWrite(ledpins[k], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
delay(t);
digitalWrite(ledpins[k-4], LOW);
delay(t);
digitalWrite(ledpins[k], HIGH);
}
}
///////////////////////////////////////////////////////////////////////////////////Effect 7
void effect_7()
{
for(int j=0; j<max_led+1; j++){
digitalWrite(ledpins[j], HIGH);
delay(t);
digitalWrite(ledpins[j+2], LOW);
delay(t);
}
for(int k = max_led+1; k>0; k--){
digitalWrite(ledpins[k], HIGH);
delay(t);
digitalWrite(ledpins[k+2], LOW);
delay(t);
}
}
void loop(){
effect_1();
effect_1();
effect_2();
effect_2();
effect_3();
effect_3();
effect_4();
effect_4();
effect_5();
effect_5();
effect_6();
effect_6();
effect_7();
effect_7();
}
The code above is for special effect for Arduino boards and ESP32 boards. You can connect your LEDs and check out the special effects of the connected LEDs in them. Have fun!
Troubleshooting Common Issues
If your LED isn’t blinking, don’t worry. Here are some common mistakes to check:
- Loose Connections: Ensure all jumper wires are securely plugged in.
- Polarity of LED: Double-check the anode and cathode.
- Wrong Code or Port: Ensure your code is error-free and that you’ve selected the correct port in the Arduino IDE.
- Damaged Components: Test with another LED or resistor if nothing works.
Why a Resistor is Essential
Without a resistor, you risk burning out your LED. The resistor limits the current flowing through the LED, protecting it and your Arduino. A 220-ohm resistor is ideal for most LEDs.
Read Also Arduino-Based Robotics Projects: From Beginner to Robotics Genius
Understanding Digital Pins
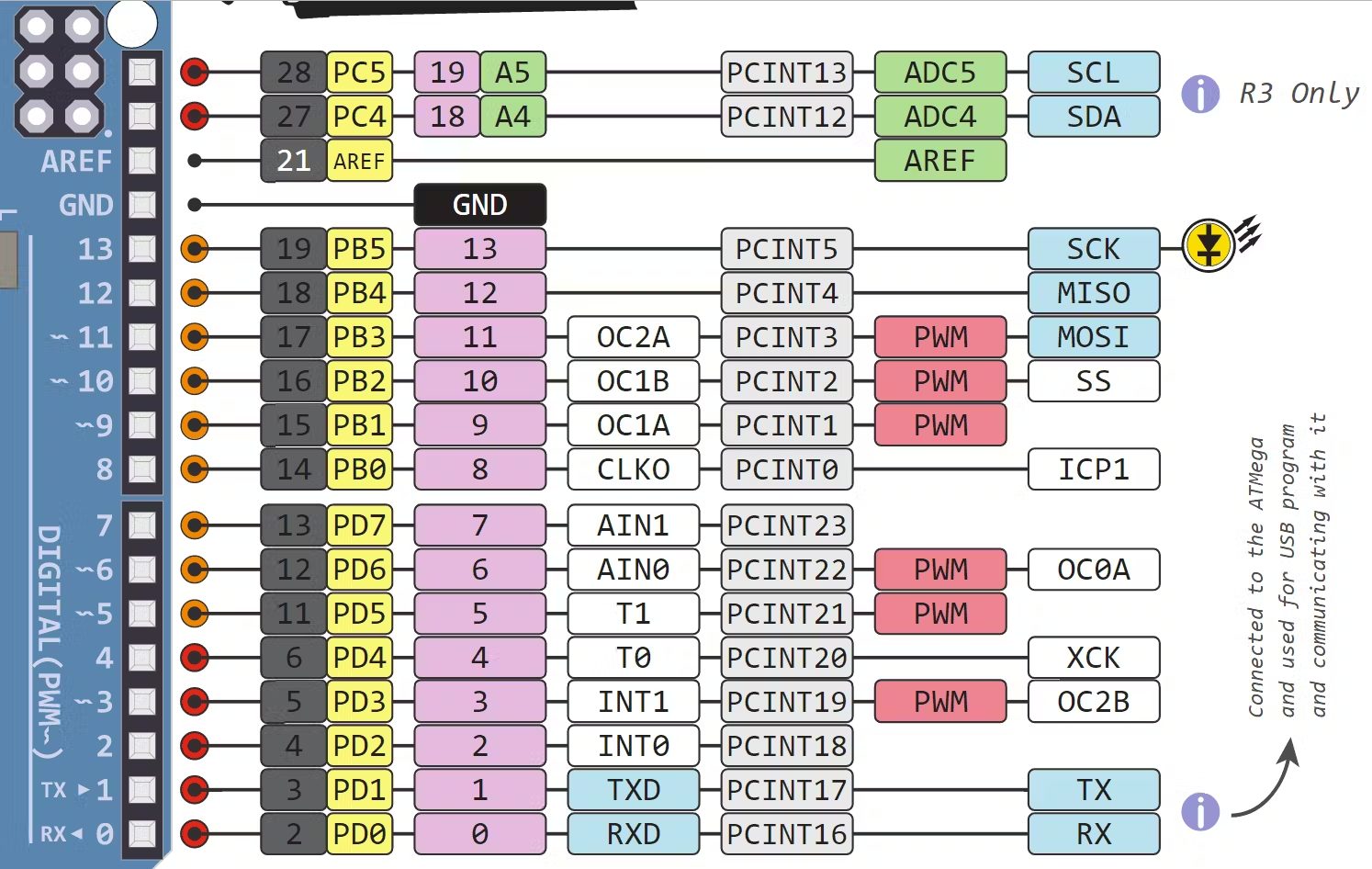
Arduino’s digital pins can be used for input or output. In this project, we used pin 13 as an output to send voltage to the LED. Understanding how digital pins work is crucial for more advanced projects.
Beyond the Basics: Expanding Your Knowledge
Once you’ve mastered the blinking LED, the Arduino world is your oyster. You can move on to projects like:
Real-World Applications of Blinking LEDs
Blinking LEDs aren’t just for learning—they’re used in real-world applications like:
- Status indicators in electronics.
- Visual feedback in user interfaces.
- Safety lights on vehicles and equipment.
Tips for Arduino Beginners
- Take Notes: Document your setups and code tweaks.
- Stay Curious: Experiment with new components and libraries.
- Ask for Help: Join Arduino forums or communities for guidance.
Conclusion
The blinking LED project is more than a beginner’s exercise; it’s your gateway into the vast world of Arduino and electronics. By mastering this simple project, you’ll gain confidence and build a solid foundation for more advanced endeavors. So, what are you waiting for? Grab your Arduino kit, and let’s make some LEDs blink!
FAQs
Can I use a different pin for the LED?
Yes, you can use any digital pin. Just update the code to match the pin number you choose.
What if I don’t have a resistor?
While it’s possible to blink the LED without a resistor, doing so may damage the LED or your Arduino. Always use a resistor for safety. But you can still proceed to blinking your LED without a resistor. There is also an Arduino code to use the internal resistors of the Arduino chip too.
Can I use multiple LEDs?
Absolutely! Connect additional LEDs to other digital pins and control them independently in your code.
What happens if I reverse the LED connections?
The LED won’t light up, but it won’t get damaged. Simply flip it around to fix the issue.
Is this project compatible with all Arduino boards?
Yes, the blinking LED project works with any Arduino board, including Uno, Nano, and Mega.